The Best Web Scraping API to Avoid Getting Blocked
The ScrapingBee web scraping API handles headless browsers, rotates proxies for you, and offers AI-powered data extraction.
Render your web page as if it were a real browser.
We manage thousands of headless instances using the latest Chrome version. Focus on extracting the data you need, not dealing with inefficient headless browsers.
ScrapingBee simplified our day-to-day marketing and engineering operations a lot . We no longer have to worry about managing our own fleet of headless browsers, and we no longer have to spend days sourcing the right proxy providerMike Ritchie CEO @ SeekWell
Render JavaScript to scrape any website.
With JavaScript rendering, a simple parameter enables you to scrape any web page, even single-page applications using React, AngularJS, Vue.js, or any other libraries.
You can also build custom scraping requests or scripts with ScrapingBee to handle complex JavaScript scenarios and tailor the scraping process to your needs.
ScrapingBee is helping us scrape many job boards and company websites without having to deal with proxies or chrome browsers. It drastically simplified our data pipelineRussel Taylor CEO @ HelloOutbound
Rotate proxies to
bypass rate limiting.
Thanks to our large proxy pool, you can bypass rate limiting while scraping web pages, hiding your bots and reducing the chances of being blocked.
ScrapingBee clear documentation, easy-to-use API, and great success rate made it a no-brainer.Dominic Phillips Co-Founder @ CodeSubmit
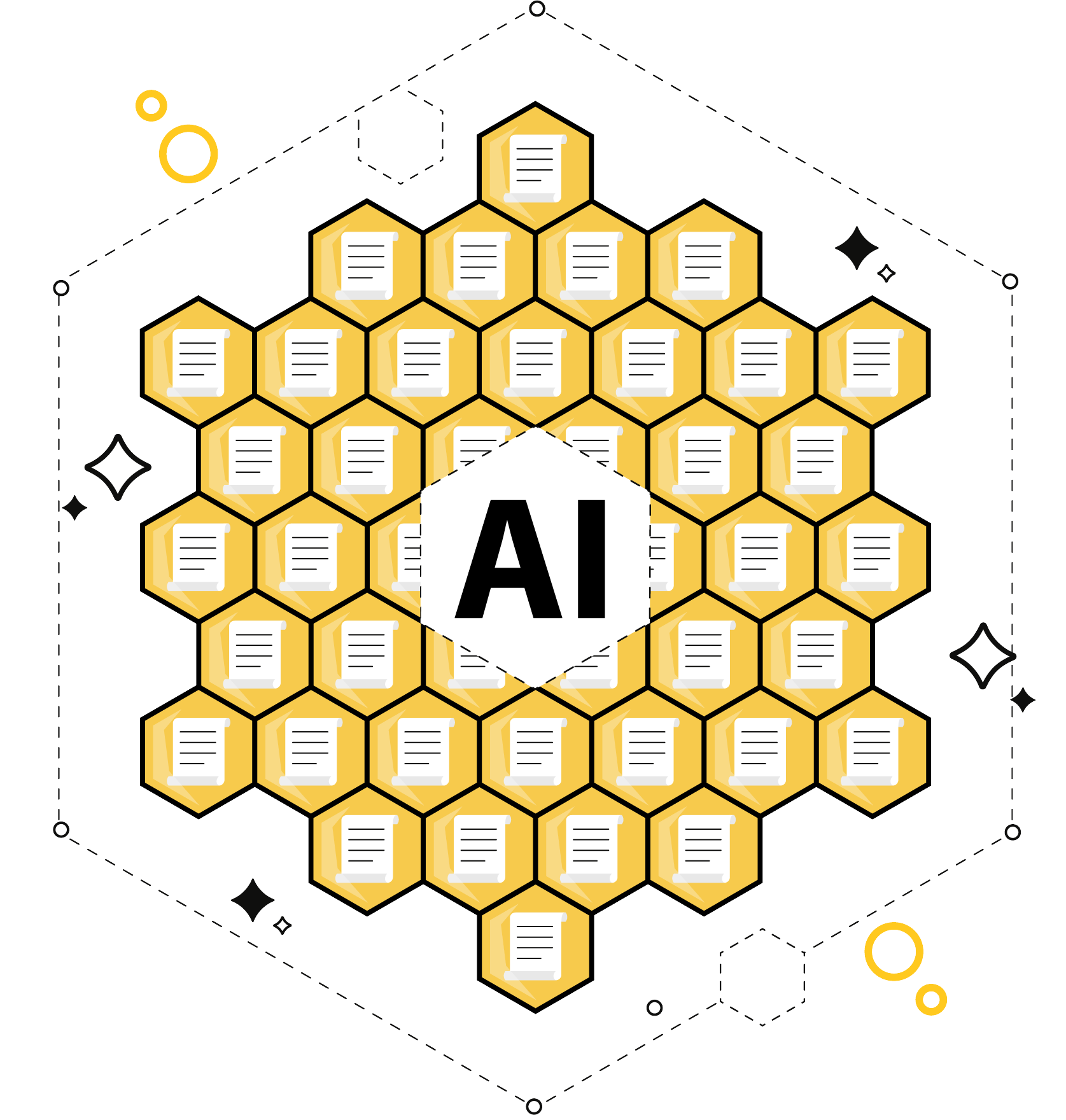
Use the power of AI web scraping.
With plain English, you simply just describe the data you want extracted, and let the AI platform identify the relevant content for you and return it as a structured data output.
ScrapingBee's AI web scraping provides detailed information extraction, allowing users to obtain comprehensive details and accurate information from web pages.
Scraping is 50% dealing with ever-changing HTML files and 50% massaging data into a useable format. ScrapingBee’s incredible AI feature can do both much better than I ever could. Now I can spend 100% of my time on what matters most; my business.Arvid Kahl Founder @ Podscan
Six ways to use ScrapingBee for web harvesting
Wondering how our customers use our web scraping API?
From a general web scrape to JavaScript rendering, our simple API does it all.
ScrapingBee also offers a range of scraping APIs designed for different data extraction needs.
1. General Web Scraping
ScrapingBee web scraping API works great for general web scraping tasks like real estate scraping, price-monitoring, extracting reviews without getting blocked.
2. Data Extraction
Getting HTML is cool, getting formatted JSON data is better. Thanks to our easy-to-use extraction rules, get just the data you need with one simple API call.
3. JavaScript scenario
If you need to click, scroll, wait for some elements to appear or just run some custom JavaScript code on the website you want to scrape, check our JS scenario feature.
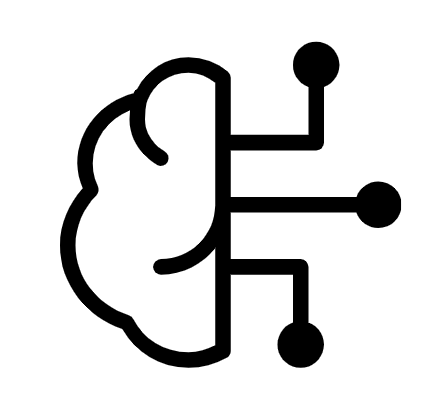
4. AI web scraping
Leverage our AI web scraping feature to extract the right content by just expressing what you need in plain English without using CSS selectors!
5. Screenshots
Need a screenshot of that website and not HTML? You can do this very easily with our screenshot feature. We also support full page and partial screenshots!
6. Search Engine Result Page
Scraping search engine result pages is extremely painful because of rate limits. Thanks to our Google search API, it's now easier than ever.
You're in great company.
2,500+ customers all around the globe use ScrapingBee to solve their web scraping needs.
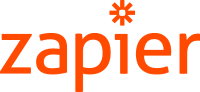
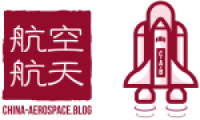
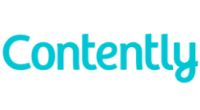
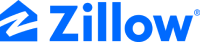
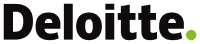
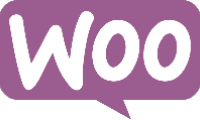
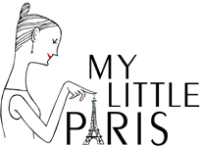
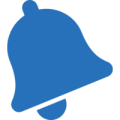
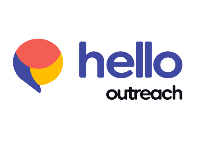

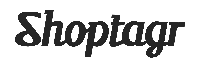
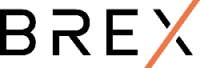
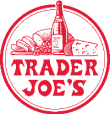
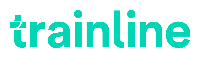
Simple, transparent pricing.
Cancel anytime, no questions asked!
Need more credits and concurrency per month?
Not sure what plan you need? Try ScrapingBee with 1000 free API calls.
(No credit card required)
Developers are asking...
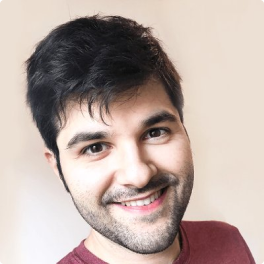
Kevin is a web scraping expert and author of The Java Web Scraping Handbook. He's been involved in many web scraping projects, for banks, startups, and E-commerce stores. He now handles all the marketing at ScrapingBee.
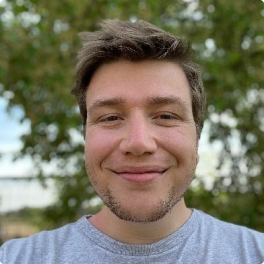
Pierre is a data-engineer. He's been involved in many startups, in the US and in Europe. Previously, with Kevin, he co-founded PricingBot a price-monitoring service for E-commerce. He now takes care of the tech / product side of ScrapingBee.
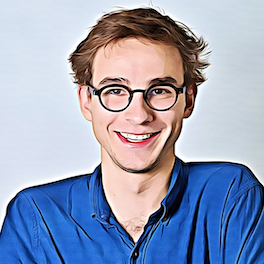
Etienne is a senior developer with a wide range of experiences. From developing a product from the ground-up at a fast-scaling startup to computer vision for the aerospace industry, he's now in charge of everything technical at ScrapingBee. He also gives some help with the trickiest support tickets.
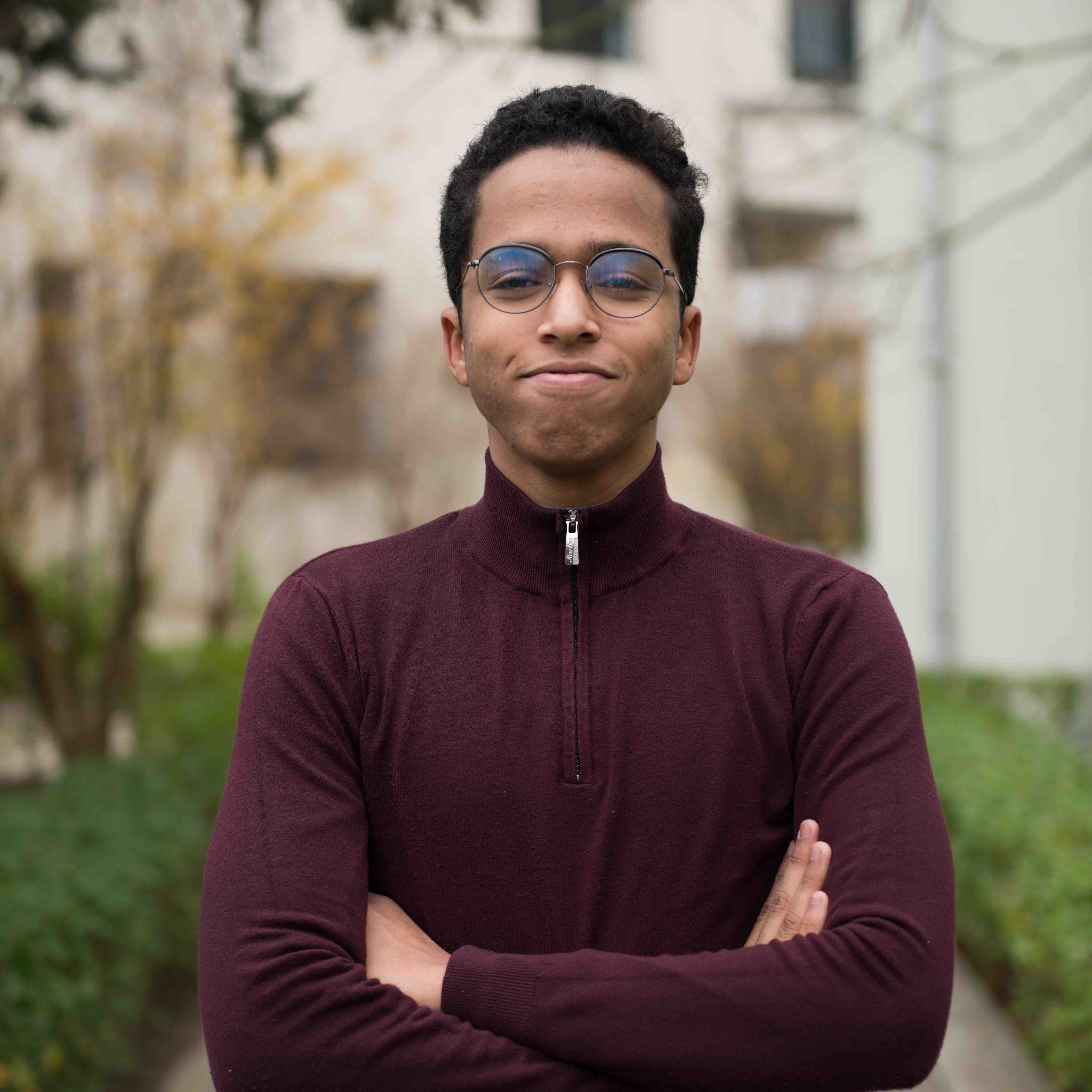
Nizar is an experienced support engineer who will go above and beyond to help with your ScrapingBee experience. Having a wide range of technical skills, he will help you fix your scraping scripts and understand how you can extract the data you need with ScrapingBee.